Mastering PHP Basics: A Comprehensive Guide for Beginners
As the backbone of many websites and web applications, PHP (Hypertext Preprocessor) stands as one of the most popular server-side scripting languages in the world of web development. Whether you're a novice looking to kickstart your journey in programming or an experienced developer aiming to expand your skill set, mastering PHP basics is an essential step towards becoming proficient in web development.
Understanding the Importance of PHP Basics
Before we dive into the intricacies of PHP syntax and programming, let's first understand why mastering PHP basics is crucial for aspiring web developers. PHP is renowned for its versatility and ease of use, making it an ideal choice for building dynamic websites and web applications. By learning PHP basics, you gain the foundational knowledge needed to create interactive web experiences, process user input, and communicate with databases seamlessly.
What You'll Learn in This Guide
Our comprehensive guide is designed to equip you with the essential skills and knowledge required to master PHP basics. Here's a sneak peek into what you can expect to learn:
- PHP Language Basics: We'll start from scratch, covering everything from basic syntax to advanced programming concepts. Whether you're familiar with other programming languages or entirely new to coding, our step-by-step approach ensures that you grasp each concept thoroughly.
- Understanding PHP Syntax: Dive deep into the syntax of PHP, including variables, constants, operators, and expressions. You'll learn how to write clean and efficient code that adheres to best practices, setting the foundation for building robust web applications.
- Handling Data Types: Master the art of working with different data types in PHP, including strings, numbers, doubles, booleans, and null values. Understanding data types is essential for manipulating data effectively and ensuring the accuracy of your web applications.
- Working with Control Structures: Learn how to implement conditional statements and looping constructs in PHP to control the flow of your code. Whether you need to execute code based on certain conditions or iterate through arrays, mastering control structures is key to writing dynamic and responsive web applications.
- Managing Arrays and Functions: Explore the power of arrays in PHP and discover how they can simplify data manipulation tasks. Additionally, learn the basics of defining functions, passing parameters, and organizing your code for maximum reusability and maintainability.
Why Choose PHP: The Right Way?
With a plethora of programming languages and frameworks available, you might wonder why PHP stands out as the preferred choice for many web developers. PHP: The Right Way offers a compelling answer to this question. It serves as an easy-to-read, quick reference guide for adhering to popular PHP coding standards and best practices.
Next Steps
Now that you understand the importance of mastering PHP basics and what to expect from this guide, it's time to roll up your sleeves and embark on your learning journey. In the next section, we'll delve into the core concepts of PHP language basics, laying the groundwork for your proficiency in web development.
Object-oriented PHP: Elevating Your Skills
Now, let's embark on a journey into the realm of object-oriented PHP. Object-oriented programming (OOP) is a paradigm that allows developers to model real-world entities as objects, each with its properties and behaviors. PHP fully supports OOP principles, enabling you to write more modular, reusable, and maintainable code.
Classes and Objects:
At the heart of OOP in PHP are classes and objects. A class serves as a blueprint for creating objects, and defining their structure and behavior. Let's create a simple class representing a Car:
PHP copy code class Car {
// Properties
public $brand;
public $model;
public $year;
// Constructor
public function __construct($brand, $model, $year) {
$this->brand = $brand;
$this->model = $model;
$this->year = $year;
}
// Method
public function start() {
return $this->brand . " " . $this->model . " started.";
}
}
// Create an object
$car = new Car("Toyota", "Corolla", 2022);
// Accessing object properties
echo $car->start(); // Outputs: Toyota Corolla started.
Inheritance and Polymorphism:
Inheritance allows classes to inherit properties and methods from other classes, promoting code reuse and extensibility. Let's extend our Car class to create a LuxuryCar class:
PHP copy code class LuxuryCar extends Car {
// Additional properties
public $features;
// Constructor
public function __construct($brand, $model, $year, $features) {
parent::__construct($brand, $model, $year);
$this->features = $features;
}
// Method override
public function start() {
return "Starting " . $this->brand . " " . $this->model . " with luxury features.";
}
}
// Create an object
$luxuryCar = new LuxuryCar("BMW", "X5", 2022, "Leather seats, Sunroof");
// Accessing overridden method
echo $luxuryCar->start(); // Outputs: Starting BMW X5 with luxury features.
Polymorphism allows objects of different classes to be treated as objects of a common superclass, facilitating code flexibility and scalability.
Encapsulation and Abstraction:
Encapsulation involves bundling data and methods within a class, hiding the internal implementation details from the outside world. Abstraction focuses on defining clear interfaces for interacting with objects, allowing users to utilize objects without needing to understand their internal complexities.
Object-oriented Design Principles:
In addition to the core OOP concepts mentioned above, mastering object-oriented PHP involves understanding and applying design principles such as SOLID (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion). These principles guide developers in writing clean, maintainable, and scalable code.
Conclusion: Embrace the Power of Object-oriented PHP
Congratulations on completing our exploration of object-oriented PHP! You've unlocked the potential to write more modular, reusable, and scalable code using OOP principles. By mastering classes, objects, inheritance, encapsulation, and abstraction, you're well-equipped to tackle complex PHP projects with confidence.
As you continue your journey in PHP development, remember to practice regularly, explore advanced topics, and stay updated on industry best practices. Whether you're building web applications, APIs, or software solutions, object-oriented PHP will be your ally in creating robust and efficient solutions.
Stay tuned for our upcoming articles, where we'll dive deeper into advanced PHP techniques, frameworks, and real-world applications. Until then, keep coding, exploring, and pushing the boundaries of what you can achieve with PHP.
Happy coding!
Frequently Asked Questions
What are the core concepts covered in this PHP basics course?
Our PHP basics course covers essential concepts such as PHP syntax, variables, operators, data types, arrays, functions, error handling, file handling, and more.
Is prior programming experience required to learn PHP?
No prior programming experience is required. Our course is designed for beginners and includes step-by-step instructions, practical exercises, and quizzes to reinforce learning.
How will I benefit from learning PHP basics?
Learning PHP basics opens up opportunities to develop dynamic websites, web applications, and server-side scripts. It lays a solid foundation for pursuing advanced PHP programming and other web development technologies.
What resources are provided to help me learn PHP basics?
Our course offers a combination of video tutorials, hands-on exercises, assignments, quizzes, and supplementary reading materials. Additionally, our instructors provide guidance and support to help you succeed.
Is this course suitable for experienced developers?
While our PHP basics course is beginner-friendly, experienced developers can also benefit from it as a refresher or to fill in any knowledge gaps. Advanced topics are covered in subsequent courses.
Insights from our team
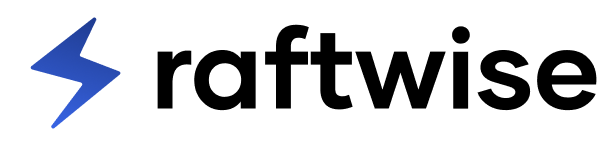
The only tool you need
to engage and grow on Linkedin!
Generate Linkedin content in minutes
- Subscribe at only $1.99/month for the first 3 months
- Early access to all the new features
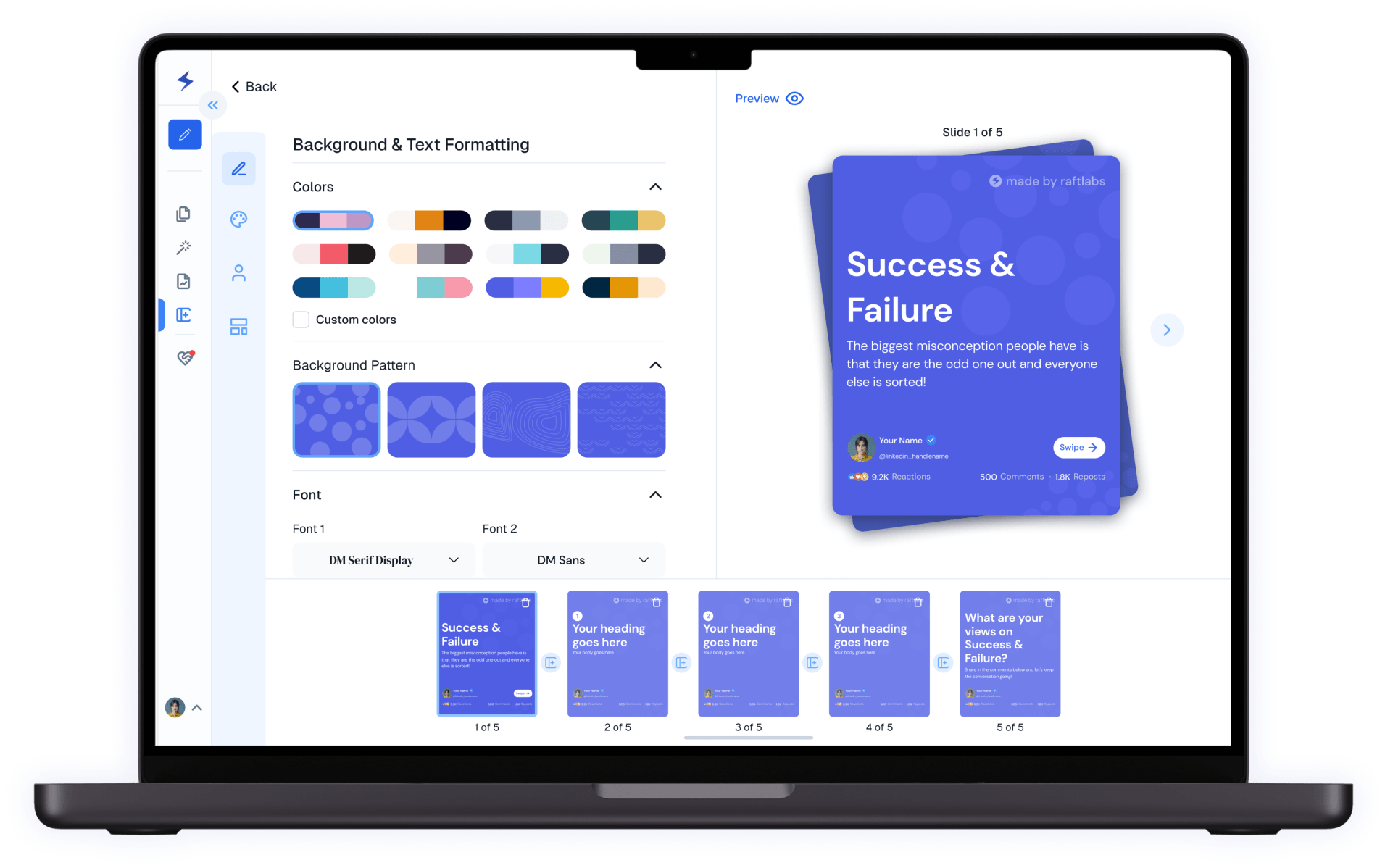
Ready to build
something amazing?
With experience in product development across 24+ industries, share your plans,
and let's discuss the way forward.